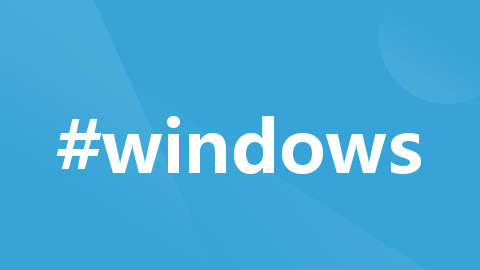
函数编程实践
这样就ok了,通过Function实现了一些自定义过滤,当然也可以很复杂。比如我要实现,两个集合中的对应位置的元素是否相等(相等的条件我自己定义)复制一点源码,可以看到,入参为三个参数,调用apply 返回指定结果R。复制一点源码可以看到,入参为两个 调用apply 返回给定结果R。比如我要实现: list中的元素的id的提取,下面为测试代码。具体代码,在我的gitee 中 test项目中。函数编
·
Function Bifunction实践
函数编程中的两个东西 Function
@FunctionalInterface
public interface Function<T, R> {
/**
* Applies this function to the given argument.
*
* @param t the function argument
* @return the function result
*/
R apply(T t);
复制一点源码可以看到,入参为两个 调用apply 返回给定结果R
BiFunction
@FunctionalInterface
public interface BiFunction<T, U, R> {
/**
* Applies this function to the given arguments.
*
* @param t the first function argument
* @param u the second function argument
* @return the function result
*/
R apply(T t, U u);
复制一点源码,可以看到,入参为三个参数,调用apply 返回指定结果R
具体要怎么使用呢?举两个栗子:
1 Function
比如我要实现: list中的元素的id的提取,下面为测试代码
@Test
void 测试函数() {
List<Tree> trees = initList();//初始化一些集合 随意
List<String> ids = Lists.newArrayList();
trees.forEach(tree -> {
ApplyFunction applyFunction = new ApplyFunction(Tree::getId);//真正执行的方法
ids.add(applyFunction.getGetId().apply(tree));// 执行入口
});
ids.forEach(s -> System.out.println(s));
}
粘贴出ApplyFunction 代码
@Data
public class ApplyFunction {
private Function<Tree,String> getId;
public ApplyFunction(Function<Tree,String> getId) {
this.getId = getId;
}
}
这样就ok了,通过Function实现了一些自定义过滤,当然也可以很复杂
2 Bifunction
比如我要实现,两个集合中的对应位置的元素是否相等(相等的条件我自己定义)
下面为测试代码
@Autowired
EqualsFunction equalsFunction;
@Test
void 是否一致() {
List<Tree> trees = initList();
List<Tree> trees1 = initList1();
int size = trees.size();
if (trees.size()>trees1.size()) {
size = trees1.size();
}
for (int i = 0; i < size; i++) {
Tree tree = trees.get(i);
Tree tree1 = trees1.get(i);
Boolean equal = (Boolean) equalsFunction.isEqual(tree, tree1);
System.out.print(JSONUtil.toJsonStr(tree));
System.out.print(JSONUtil.toJsonStr(tree1));
System.out.println(equal);
}
}
粘贴下打印结果
{"pid":"root","id":"0"}{"pid":"root","id":"0"}true
{"pid":"0","id":"1"}{"pid":"0","id":"1"}true
{"pid":"0","id":"2"}{"pid":"10","id":"2"}false
{"pid":"2","id":"6"}{"pid":"12","id":"6"}false
{"pid":"6","id":"7"}{"pid":"16","id":"7"}false
{"pid":"1","id":"3"}{"pid":"11","id":"3"}false
{"pid":"1","id":"4"}{"pid":"11","id":"4"}false
{"pid":"4","id":"5"}{"pid":"4","id":"5"}true
{"pid":"6","id":"7"}{"pid":"6","id":"7"}true
{"pid":"4","id":"5"}{"pid":"4","id":"5"}true
粘贴另外两个类EqualsConfig和EqualsFunction
public class EqualsFunction<P extends Tree,S extends Tree>{
private BiFunction<Tree, Tree, Boolean> equal;
public EqualsFunction(BiFunction<Tree, Tree, Boolean> equal) {
this.equal = equal;
}
public Object isEqual(Tree tree, Tree tree1){
Boolean apply = equal.apply(tree, tree1);
return apply;
}
}
@Component
public class EqualsConfig{
@Bean
public EqualsFunction registerEqualsFunction(){
return new EqualsFunction<>((tree1,tree2)-> ObjectUtils.equals(tree1.getId(),tree2.getId()) && ObjectUtils.equals(tree1.getPid(),tree2.getPid()));
}
}
至此,完成两个类的比较
具体代码,在我的gitee 中 test项目中
https://gitee.com/flgitee/test
更多推荐
所有评论(0)