java中数据表中简单的类一对多关系
查询表中的所有雇员信息,以及查询每个员工的部门信息和领导信息/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the templat
·
查询表中的所有雇员信息,以及查询每个员工的部门信息和领导信息
注意看返回值的类型
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package testdemo;
/**
*
* @author Jone
*/
class emp{
private int empno;
private String ename;
private String job;
private double sal;
private double comm;
private emp mgr;
private Dept dept;
public emp(){};
public emp(int empno, String ename, String job, double sal, double comm){
this.empno = empno;
this.job = job;
this.comm = comm;
this.ename = ename;
this.sal = sal;
}
public void setMgr(emp mgr){
this.mgr = mgr;
}
public emp getMgr(){
return this.mgr;
}
public void setDept(Dept dept){
this.dept = dept;
}
public Dept getDept(){
return this.dept;
}
public void setEmpno(int empno){
this.empno = empno;
}
public void setEname(String ename)
{
this.ename = ename;
}
public void setJob(String job){
this.job = job;
}
public void setSal (double sal){
this.sal = sal;
}
public void setComm (double comm){
this.comm = comm;
}
public int getEmpno(){
return this.empno;
}
public String getEname(){
return this.ename;
}
public String getJob(){
return this.job;
}
public double getSal(){
return this.sal;
}
public double getComm(){
return this.comm;
}
public String getEmpInfo(){
return "empno = "+ this.empno +
"ename = "+ this.ename +
"job = "+ this.sal +
"sal = " + this.comm ;
}
}
class Dept{
private int deptno;
private String dname;
private String loc;
private emp [] Emps;
public Dept(){}
public Dept(int deptno, String dname, String loc){
this.deptno = deptno;
this.dname = dname;
this.loc = loc;
}
public void setEmps(emp [] Emps){
this.Emps = Emps;
}
public emp[] getEmps(){
return this.Emps;
}
public void setDeptno(int deptno){
this.deptno = deptno;
}
public void setDnama(String dname){
this.dname = dname;
}
public void setLoc(String loc){
this.loc = loc;
}
public int getDeptno(){
return this.deptno;
}
public String getDname(){
return this.dname;
}
public String getLoc(){
return this.loc;
}
public String gerDeptInfo(){
return "deptno = "+ this.deptno +
"dname = "+ this.dname +
"lco = " + this.loc;
}
}
public class TestDemo {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
//第一步设置类对象间的关系
//1. 分别创建各自类的对象
Dept dept = new Dept(10,"Accontiing ", "Newyork");
emp ea = new emp(7369, "smith", "clerck", 800.0, 0.0);
emp eb = new emp(7366, "allen", "manager", 2450.0, 0.0);
emp ec = new emp(7777, "king", "preseident", 5000.0,0.0);
//2. 设置雇员的关系
ea.setMgr(eb);
eb.setMgr(ec);
//3设置雇员和部门的关系
ea.setDept(dept);
eb.setDept(dept);
ec.setDept(dept);
//3设置部门与雇员的关系
dept.setEmps(new emp[] {ea,eb,ec});
//第二步进行数据的取得
//输出一部门的全部雇员
System.out.println(dept.gerDeptInfo());
for(int i=0; i<dept.getEmps().length; i++)
{
System.out.println(dept.getEmps() [i].getEmpInfo());
if(dept.getEmps()[i].getMgr() != null) //输出雇员的领导
System.out.println(dept.getEmps() [i].getMgr().getEmpInfo());
}
System.out.println("=======================\n");
System.out.println(ea.getEmpInfo());
if(ea.getMgr() != null)
System.out.println(ea.getMgr().getEmpInfo());
if(ea.getDept() != null)
System.out.println(ea.getDept().gerDeptInfo());
}
}
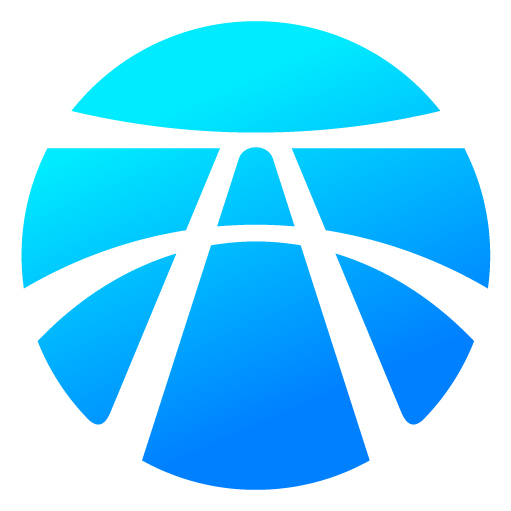
开放原子开发者工作坊旨在鼓励更多人参与开源活动,与志同道合的开发者们相互交流开发经验、分享开发心得、获取前沿技术趋势。工作坊有多种形式的开发者活动,如meetup、训练营等,主打技术交流,干货满满,真诚地邀请各位开发者共同参与!
更多推荐
所有评论(0)