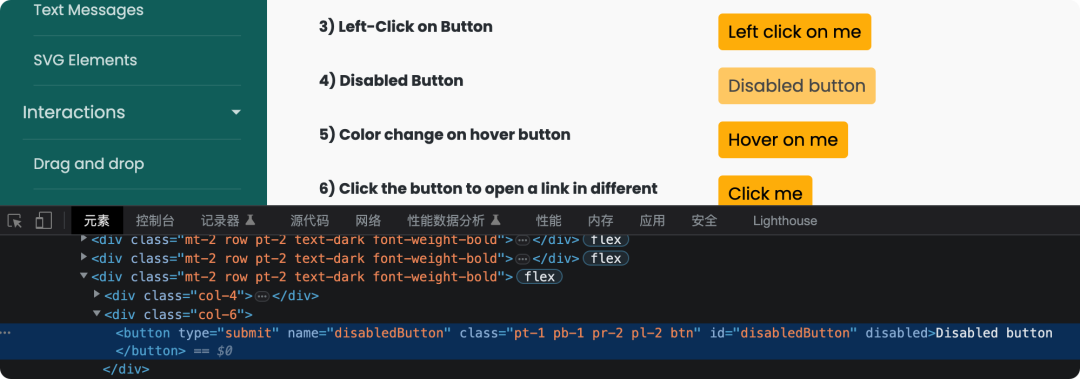
新一代最强开源UI自动化测试神器Playwright(Java版)六(断言)
Playwright是一个流行的UI自动化测试框架,用于编写UI自动化测试。在测试中,断言是一个非常重要的概念,用于验证测试的结果是否符合预期。Playwright提供了一些内置的断言函数,可以帮助测试人员编写更加简洁和可读的测试代码。本文将介绍Playwright中的断言函数,并提供一些示例,以帮助您更好地理解如何使用这些函数来编写高质量的自动化测试。LocatorAssertions类提供断言
Playwright
是一个流行的UI
自动化测试框架,用于编写UI自动化测试。在测试中,断言是一个非常重要的概念,用于验证测试的结果是否符合预期。Playwright
提供了一些内置的断言函数,可以帮助测试人员编写更加简洁和可读的测试代码。本文将介绍Playwright
中的断言函数,并提供一些示例,以帮助您更好地理解如何使用这些函数来编写高质量的自动化测试。LocatorAssertions
类提供断言方法,可用于对测试中的定位器状态进行断言。
PART 01 断言复选框是否被选中
// 点击勾选
page.getByLabel("abcd").check();
// 断言 abcd 复选框是否已选中,若未选择则抛出异常
// Exception in thread "main" org.opentest4j.AssertionFailedError: Locator expected to be checked
assertThat(page.getByLabel("abcd")).isChecked();
PART 02 断言元素是否被启用或禁用
// 元素是否被启用,若未启用,则抛出异常Exception in thread "main" org.opentest4j.AssertionFailedError: Locator expected to be enabled
assertThat(page.locator("#disabledButton")).isEnabled();
// 元素是否被禁用
//assertThat(page.locator("#disabledButton")).isDisabled();
PART 03 断言元素是否可编辑
assertThat(page.locator("body > label:nth-child(2) > input[type=textbox]")).isEditable();
PART 04 断言元素是否为空
// 元素是否为空,若不为空,则抛出异常
// Exception in thread "main" org.opentest4j.AssertionFailedError: Locator expected to be empty
assertThat(page.locator("//*[@id='newName']")).isEmpty();
PART 05 断言元素是否被聚焦
如光标是否显示在输入框内
// 点击元素,使其光标在输入框内
page.locator("body > label:nth-child(2) > input[type=textbox]").click();
// 元素是否被聚焦(如光标是否在输入框内),若不在,抛出异常
// Exception in thread "main" org.opentest4j.AssertionFailedError: Locator expected to be focused
assertThat(page.locator("body > label:nth-child(2) > input[type=textbox]")).isFocused();
PART 06 断言元素是否可见
// 校验元素是否可见
assertThat(page.locator("#uv")).isVisible();
// 点击 Visibility hidden 按钮
page.getByRole(AriaRole.BUTTON, new Page.GetByRoleOptions().setName("Visibility hidden")).click();
// 校验元素是否隐藏
assertThat(page.locator("#uv")).isHidden();
PART 07 断言元素是否包含指定文本
确保定位器指向包含给定文本的元素。也可以对值使用正则表达式。如果将数组作为期望值传递,则期望值是:
-
定位器解析为元素列表。
-
此列表子集中的元素分别包含预期数组中的文本。
-
元素的匹配子集与预期数组具有相同的顺序。
-
预期数组中的每个文本值都与列表中的某个元素匹配。
<ul>
<li>Item Text 1</li>
<li>Item Text 2</li>
<li>Item Text 3</li>
</ul>
// ✔️ 以正确的顺序包含正确的元素
assertThat(page.locator("ul > li")).containsText(new String[] {"Text 1", "Text 2", "Text 3"});
// ❎ 错误的顺序,抛出异常
// Exception in thread "main" org.opentest4j.AssertionFailedError: Locator expected to contain text: [Text 3, Text 2]
// Received: [Item Text 1, Item Text 2, Item Text 3]
assertThat(page.locator("ul > li")).containsText(new String[] {"Text 3", "Text 2"});
// ❎ 不包含此元素,抛出异常
// Exception in thread "main" org.opentest4j.AssertionFailedError: Locator expected to contain text: [TesterRoad]
// Received: [Item Text 1, Item Text 2, Item Text 3]
assertThat(page.locator("ul > li")).containsText(new String[] {"TesterRoad"});
// ✔️ 定位器指向外部列表元素,而不是li
assertThat(page.locator("ul")).containsText(new String[] {"Text 3"});
PART 08 断言元素是否包含指定属性
// 断言 input 元素是否包含value属性,属性的值为 Tyto
assertThat(page.locator("//*[@id='newName']")).hasAttribute("value","Tyto");
PART 09 断言元素是否有class属性
<div class='selected row' id='component'>公众号:测试工程师成长之路</div>
assertThat(page.locator("#component")).hasClass(Pattern.compile("selected"));
assertThat(page.locator("#component")).hasClass("selected row");
PART 10 断言父元素下子元素的数量
<ul>
<li>Item Text 1</li>
<li>Item Text 2</li>
<li>Item Text 3</li>
</ul>
// 断言ul下li的数量
assertThat(page.locator("ul > li")).hasCount(3);
PART 11 断言元素是否有CSS属性
// 断言元素的display是否为block
assertThat(page.locator("//*[@id='nestedZup']")).hasCSS("display","block");
PART 12 断言元素id属性值
assertThat(page.locator("//*[@id='newName']")).hasId("newName");
PART 13 断言元素是否有JavaScript属性
// 断言javascript src属性的值为https://mimg.127.net/external/mail-index/index-promote.js
assertThat(page.locator("body > script:nth-child(17)")).hasJSProperty("src","https://mimg.127.net/external/mail-index/index-promote.js");
PART 14 断言元素的文本
<ul>
<li>Item Text 1</li>
<li>Item Text 2</li>
<li>Item Text 3</li>
</ul>
// 断言元素的文本
assertThat(page.locator("body > ul > li:nth-child(1)")).hasText("Item Text 1");
assertThat(page.locator("body > ul > li:nth-child(1)")).hasText(Pattern.compile("Item.*"));
PART 15 断言元素的value值
// 断言value值
assertThat(page.locator("//*[@id='newName']")).hasValue("Tyto");
PART 16 断言元素多选后的值
确保定位器指向多选/组合框(即具有multiple
属性的 select
),并选择指定值。
<select id="favorite-colors" multiple>
<option value="R">Red</option>
<option value="G">Green</option>
<option value="B">Blue</option>
</select>
// 多选,执行此代码会选中 Red Green
page.locator("id=favorite-colors").selectOption(new String[] {"R","G"});
// 断言多选的值
assertThat(page.locator("id=favorite-colors")).hasValues(new Pattern[] { Pattern.compile("R"), Pattern.compile("G") });
PART 17 断言页面标题
// 以126.com为例
page.navigate("https://126.com/");
// 断言页面标题
assertThat(page).hasTitle("126网易免费邮-你的专业电子邮局");
PART 18 断言指定的URL
以126.com
为例,首页点击“忘记密码”,断言跳转后的url
// 断言跳转的url
Page page1 = page.waitForPopup(() -> {
page.frameLocator("//*[@id='loginDiv']/iframe").getByRole(AriaRole.LINK, new FrameLocator.GetByRoleOptions().setName("忘记密码")).click();
});
assertThat(page1).hasURL("https://reg.163.com/naq/findPassword?pd=mail126&pkid=QdQXWEQ#/verifyAccount");
PART 19 总结
断言是UI
自动化测试中非常重要的一环,能够帮助我们判断测试结果是否符合预期。以上就是Playwright
中断言的简单使用,仅供参考,大家在实际脚本中应该灵活应用。
整套资料获取
更多推荐
所有评论(0)